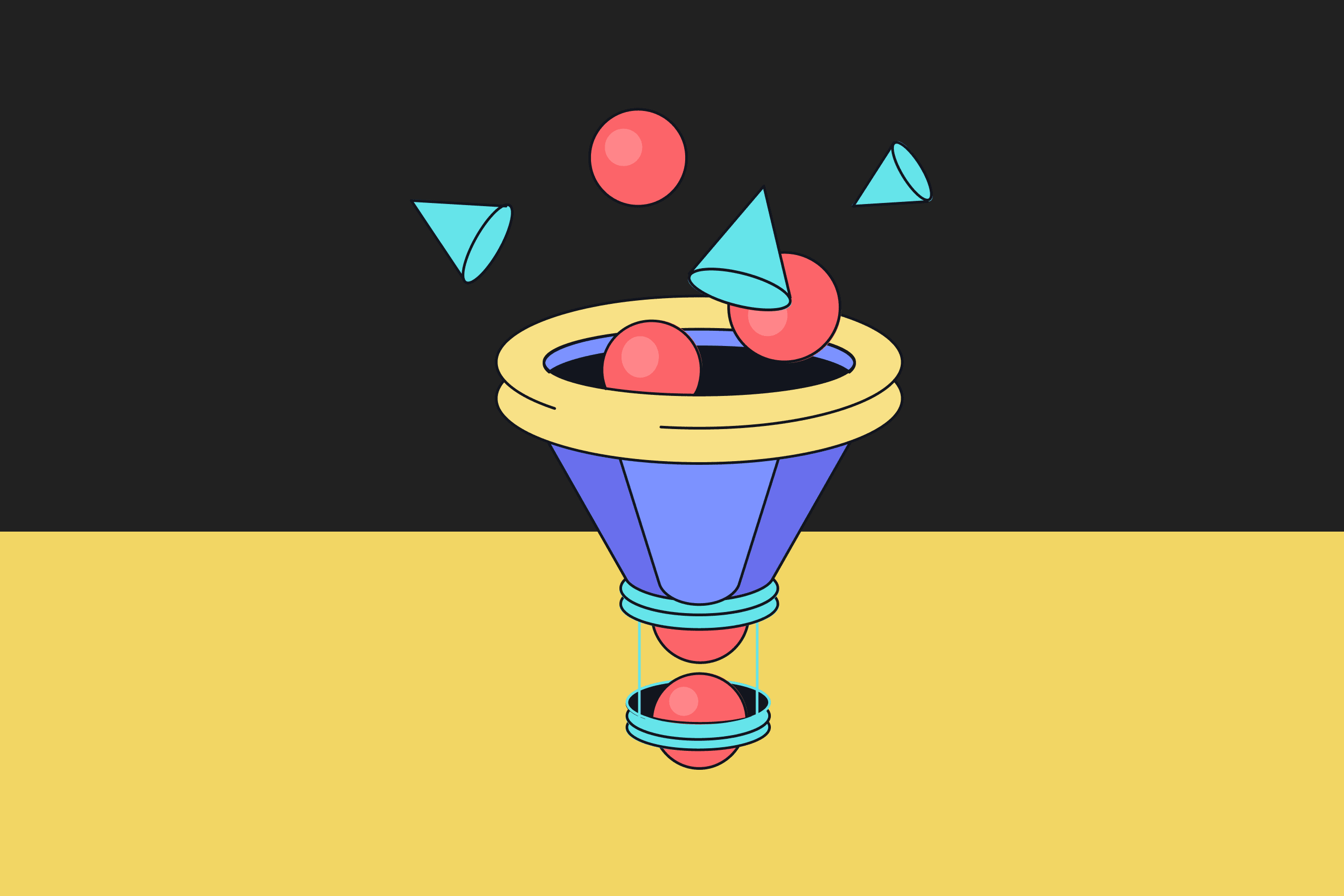
What is it like to interview at Trunk?
Coding Interviews are the worst kind of engineering interview, except for all the other kinds that are tried from time to time — Winston Churchill
Anyone who’s been through a few engineering interviews knows that they’re an ordeal. In the worst case, you’re asked strange questions with little to no relation to the position you’re interviewing for, such as how many ping pong balls fit in a school bus. Or you’re asked to write code in an unnatural manner in an unstructured text document or by hand on a whiteboard. Even in the best case, the structure of technical interviews can leave you second-guessing yourself. Was it worth it to take a shortcut to pass all the test cases, or should you have devoted more time to making the code readable? What skills mattered most to the company?
On the other hand, the high-pressure setting is hard to avoid. Companies need to rapidly gain a technical and cultural signal from you. At a startup like Trunk, everyone we bring on board is expected to contribute both in terms of writing code and participating in larger design decisions.
We’re publishing this interview guide in the interest of maximum transparency. We’d like you to enter our interview process as informed as possible, ready to show off the skills that we’re looking for and that you’ve worked so hard to acquire.
The first step in applying to work at Trunk is a recruiter screen. After that, you’ll be paired with a Trunk engineer for a one-hour technical phone screen. If things go well, we’ll bring you on for a virtual onsite. The virtual onsite will involve three one-hour technical interviews as well as a couple shorter non-technical interviews with our team members.
These interviews are discussed in more detail below.
The One-Hour Technical Interview
Your initial Technical Phone Screen (TPS) will be one hour, as will your individual technical interviews if you are brought onto the virtual onsite. They share a common structure but differ in their content.
Breakdown of a One-Hour Interview
For each interview, you’ll be paired with a Trunk engineer.
The first 10–20 minutes will be dedicated to discussing your background as well as an intro to Trunk. The interviewer may delve into your past projects and career experience so be ready to talk about it! This is also a chance for you to learn more about us.
The next 25–35 minutes are devoted to solving a technical interview over CodeSignal. Time permitting, the last few minutes will be devoted to any questions you may have about Trunk.
These are not meant to be “pass or fail” interviews but are geared more to see how you think through solving problems and how it aligns with how we do it at Trunk. We don’t do take-home tests because we respect your time — and we want to give you the opportunity to meet with more of our team and see how we work, collaborate, and ask questions.
Problem Solving
The Technical Phone Screen and one of your on-site interviews will be devoted to a programming problem, solved collaboratively via CodeSignal.
A programming language is a tool to get a job done, and we want you to put your best foot forward. The goal here is to produce clean, tested, and robust code. The programming language is your choice out of anything that CodeSignal supports — we’ve seen some uncommon ones!
That being said, we do get a stronger signal when you use typed languages, especially if you have a few years of experience. That’s not to say you can’t use vanilla JavaScript or Python if that’s what you know best, but in those cases, you should put extra care into variable naming and legibility. Remember that you’ll have the compiler/interpreter to help you, and it’s ok to look something up mid-interview if you forget!
For our backend services and frontend, we mainly use TypeScript, so this is a natural choice for these roles (and very quick to learn if you already write JavaScript!). If you’re applying for a C++ engineer role, comfort in a C-like language or a systems language like Rust or Go is a huge plus. We are looking for people who are thoughtful about expressing types and memory usage, etc. If you’re using Python, we recommend learning about type annotations and using those where appropriate.
There is nothing wrong with consulting the internet as a reference! This is a normal part of an engineer’s workflow. Questions may be ambiguous. This is by design. If you’re not sure about a detail, make sure you’re asking for clarification — clarifying ambiguity is one of your most important engineering skills!
We expect you to be solid on the fundamentals of computer science — common-in-real-life data structures and algorithms, big-O analysis, and the like — but you aren’t expected to demonstrate knowledge on anything too specific or esoteric in an interview. While algorithmic “brain teasers” were more the norm in the past, we don’t emphasize those kinds of problems.
System Design
The system design interview is an open-ended collaborative exploration of the architecture of a larger software system. You will be asked a fairly broad question (ex. “Design YouTube”) and begin to sketch out different components, guided by feedback and questions from the interviewer (note: if you’re a junior candidate with little or no experience in this area you might be asked to describe the design of a project you’ve worked on).
You’ll be expected to zoom in and out on the details of the system’s design. Nobody knows how to do everything from scratch! It’s ok to say you aren’t sure how to handle some part of the system, as long as you are conscious that this is an area you would ask someone else to help with or do some more research on.
For parts of the system that you are able to describe at a more detailed level, you should make sure that you are considering tradeoffs for each of your major decisions, and justifying them (“I will choose rate-limiting strategy X because I expect this traffic pattern, but if the pattern turned out to be quite different, then maybe I’d go with Y…).
You might think we’re giving away the game, but even if we told you the exact question we’d ask, these interviews are very much a choose-your-own-adventure and there’ll be enough meat on the question to last much longer than an hour (YouTube is fairly complex!). The keys are to stay persistent in moving through the design, evaluating and defending tradeoffs for the parts you know well, and making explicit notes of the parts you don’t.
How to prepare: For virtual interviews, we generally use CodeSignal’s “Whiteboard” feature (powered by a diagramming tool called Miro). Some candidates have positioned themselves in front of a whiteboard that’s visible to their webcam, which is a fine option as long as it’s legible. Some candidates might prefer just sketching out their thoughts textually and using a little bit of ASCII art. Whatever gets the job done! In any case, make sure you’re comfortable with your preferred method (and ok with another one in case something goes wrong).
Many engineers consider these interviews more stressful, as they spend much more time coding than doing high-level system design. Fortunately, it’s easy to practice — just choose any system and think through how it’s designed! Additionally, System Design Interview: An Insider’s Guide by Alex Xu is an excellent resource that has come out recently.
Code design
This is an object-oriented design problem, where you’ll be expected to produce either pseudocode or working code, depending on the interviewer. Take a sample application — how do you design the classes for it? When and why would you use an interface instead of an abstract class? We want to see how you’re thinking about and using inheritance, composition, encapsulation, and mutability.
To prepare, make sure you’re comfortable with an object-oriented language and be thoughtful about the class designs you are making and encountering in your regular work.
This is similar to the systems design interview in the sense that the scope of the problem may be larger than the interview, and there is a “choose your own adventure” characteristic to it. No two code design (or systems design) interviews are alike. Make sure you’re pacing yourself and moving at an appropriate place through the different parts of the problem. So if you’re designing classes for Fruit Ninja, if you’ve described a “Fruit” superclass and how it encapsulates the physics of a fruit exploding when a ninja sword slices through it, and then sketched out the specifics of this in the case of an Apple, there is little to be gained by also doing so for Grape, Papaya, Mango, etc (the exception being, as always, if the interviewer asks you to do so). Keep moving on to other parts of the problem.
Front End interview (if applicable)
During the front-end interview, you’ll be asked an open-ended question on implementing a web page based on an existing application. All of our front-end components use ReactJS, so deep knowledge of it is a big plus. Depending on your level this question can include: designing an API skeleton, building out an example Single Page Application, asking about core features within ReactJS, discussing interactions between front-end and back-end components, etc… (if you’re junior you’ll be provided with an example starter API skeleton, but are encouraged to update it).
To prepare, make sure you’re comfortable coding in JS/TS and have at least a base-level understanding of working with ReactJS. If you’re a front-end-only candidate, we don’t expect you to have a deep understanding of the full stack, but we will be diving into your knowledge of working with and communicating with back-end services.
Culture Fit
Some of your interviews will not be technical at all — we have a recruiter screen and potentially chatting with one of our co-CEOs.
Your personal elevator pitch
Also, each interview will involve a “getting to know you” portion and a chance to ask questions of the interviewer.
So…be prepared to talk about yourself!
What have been your experiences, positive or negative, with DevEx/DevOps/tooling at previous projects?
What is a challenging project you’ve worked on that forced you to learn something new and grow?
Be prepared to ask about Trunk!
Every workplace is different, and we want you to have as much context about your potential experience as possible.
We are still small and growing quickly, and every new person we bring on board will shape our culture significantly. How can you contribute? How would you like to contribute?
What makes someone a good fit for Trunk?
We’re looking for people who are willing to wear a lot of hats. We are a young company, and our engineers frequently cross project boundaries or work on unfamiliar parts of the codebase. We are relatively light on process, so we are looking for people who love collaboration but are self-motivated and able to thrive in a less structured environment.
As we are building a tool for engineers, we’re especially looking for product-minded people who care about the user and are passionate about developer experience. We love a good story about improving an engineering process or practice. Every company has go-to engineers who have all the cool scripts for managing complex or repetitive tasks within a repo. We’d like to think of adopting Trunk as being similar to adding those engineers to an organization — so who better to build it?
What is engineering like at Trunk?
Check out our blog to learn more about what kinds of problems we’re tackling!